Welcome to our deep dive into C# constructors, where we’ll unravel the intricacies of initializing objects in one of the most popular programming languages. Constructors play a fundamental role in object-oriented programming, serving as the blueprint for how instances of a class are created and initialized.
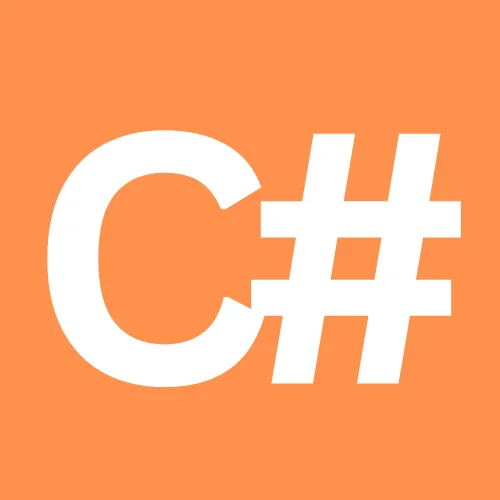
In this blog post, we’ll explore C# constructors comprehensively, covering all types and providing illustrative examples to solidify your understanding. Whether you’re a beginner eager to grasp the basics or an experienced developer seeking to refine your skills, this guide has something for everyone.
We’ll journey through the various types of constructors in C#, including default constructors, parameterized constructors, copy constructors (or their equivalent), and static constructors. Each type serves distinct purposes and offers unique capabilities, enriching your toolbox as a C# developer.
Through clear explanations and practical examples, you’ll learn how to leverage constructors effectively to initialize objects with precision, control object creation, and enhance code readability and maintainability.
So, fasten your seatbelt and get ready to embark on an enlightening exploration of C# constructors. By the end of this journey, you’ll wield the power of constructors with confidence, empowering you to build robust and efficient C# applications.
Let’s dive in and unlock the secrets of C# constructors together! ⚡
In C# programming, constructors are special methods within a class that are called when an instance of that class is created. Constructors are used to initialize the newly created object. They have the same name as the class and may have parameters to allow for different ways of initializing objects.
Here’s a basic example of a constructor in C#:
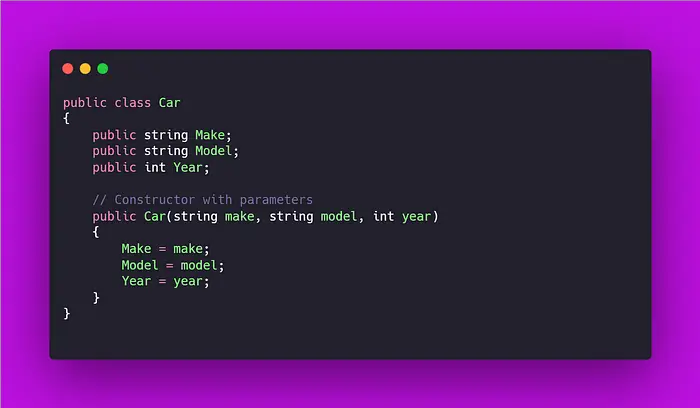
In this example, the Car
class has a constructor that takes three parameters (make
, model
, and year
). When you create a new instance of the Car
class, you can pass values for these parameters to initialize the object:
Car myCar = new Car("Toyota", "Camry", 2022);
This will create a new Car
object with the specified make, model, and year.
In C#, constructors can be divided into 5 types. Let’s Explain one by one.
#1 Default Constructor
A default constructor in C# is a constructor that is automatically provided by the compiler if no other constructors are explicitly defined in a class. It doesn’t take any parameters and initializes the fields of the class to default values. If no fields are defined, the default constructor will still be generated.
Here’s an example of a class with a default constructor:
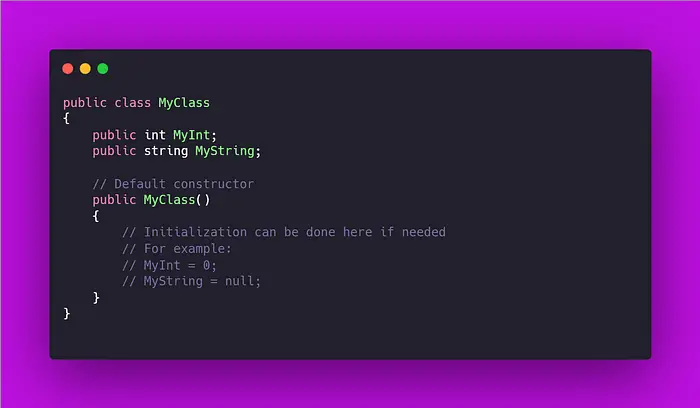
In this example, MyClass
has a default constructor. When you create an instance of MyClass
using the new
keyword without passing any parameters, the default constructor will be called automatically:
MyClass myObject = new MyClass();
This will create a new instance of MyClass
with its fields initialized to their default values (0
for MyInt
and null
for MyString
). If you explicitly define a constructor in your class, the default constructor won’t be generated unless you also explicitly define it.
#2 Parameterized Constructor
A parameterized constructor in C# is a constructor that accepts one or more parameters, allowing you to initialize the object with specific values at the time of creation. Unlike the default constructor, which initializes fields to default values, the parameterized constructor provides flexibility in initializing objects with different values based on the parameters passed.
Here’s an example of a class with a parameterized constructor:
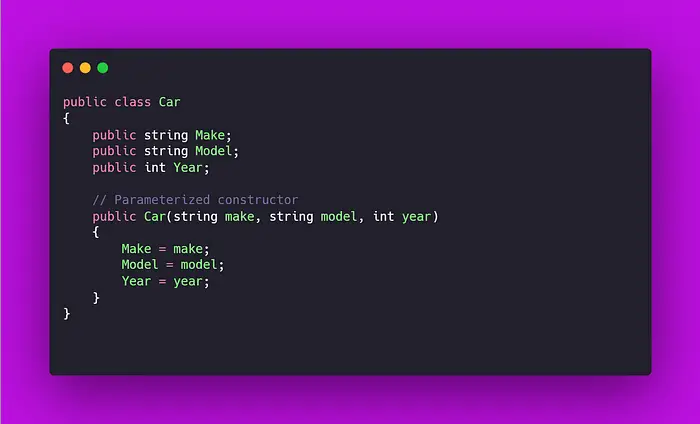
In this example, the Car
class has a parameterized constructor that takes three parameters (make
, model
, and year
). When you create an instance of Car
using this constructor, you can pass specific values for these parameters to initialize the object:
Car myCar = new Car("Toyota", "Camry", 2022);
This will create a new Car
object with the specified make (“Toyota”), model (“Camry”), and year (2022). The parameterized constructor allows you to create Car
objects with different make, model, and year values depending on the arguments you provide when constructing the object.
#3 Copy Constructor
In C#, there’s no direct support for copy constructors like in some other languages such as C++ or Java. However, you can achieve the same effect by creating a constructor that takes an instance of the same class as a parameter and initializes a new object by copying the values from the parameter object.
Here’s an example of implementing a copy constructor-like behavior in C#:
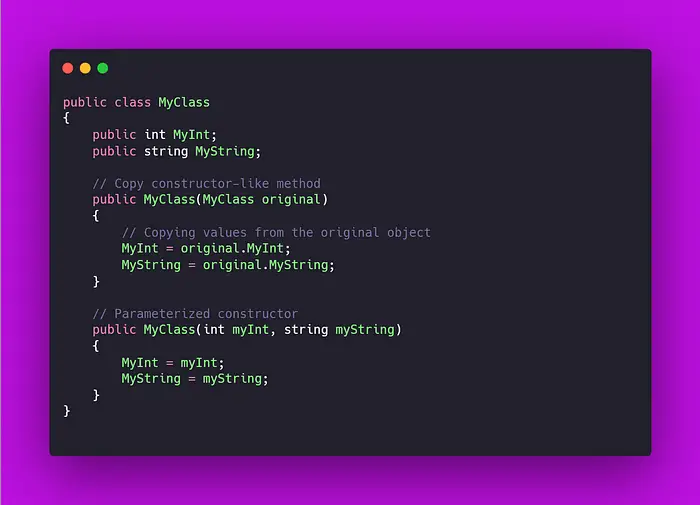
In this example, MyClass
has a parameterized constructor and a constructor that mimics a copy constructor. The copy constructor-like method MyClass(MyClass original)
takes an instance of MyClass
as a parameter and initializes a new MyClass
object by copying the values of the fields from the original object.
Here’s how you can use it:
MyClass originalObject = new MyClass(10, "Hello");
// Using the copy constructor-like method to create a new object
MyClass copiedObject = new MyClass(originalObject);
// Now, copiedObject has the same values as originalObject
Console.WriteLine($"MyInt: {copiedObject.MyInt}, MyString: {copiedObject.MyString}");
This will create a new MyClass
object (copiedObject
) with the same values as the original object (originalObject
). This mimics the behavior of a copy constructor by allowing you to create a new object initialized with the values of an existing object.
#4 Static Constructor
A static constructor in C# is a special type of constructor that is used to initialize static data members of a class or to perform any other type of initialization that needs to be done only once before any instance of the class is created or any static members are accessed.
Here’s an example of a static constructor:
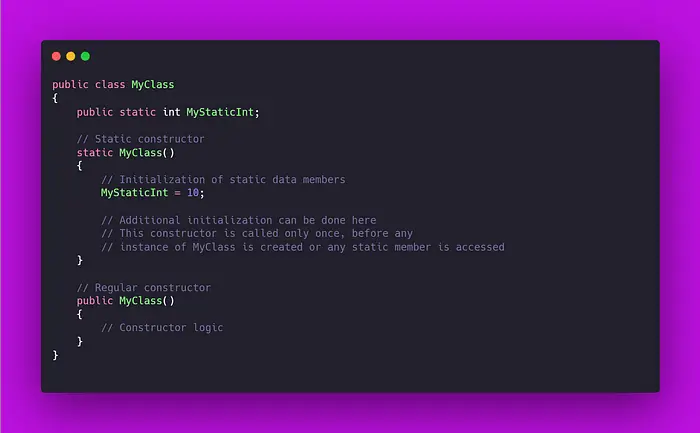
In this example, MyClass
has a static constructor (static MyClass()
). This constructor is called automatically by the runtime before any static members of the class are accessed or any instance of the class is created.
You can’t explicitly call a static constructor. The runtime ensures that it is called automatically, and it’s invoked only once per application domain. The static constructor is useful for initializing static data members or performing any other type of initialization that needs to happen at the class level before any instance is created or any static member is accessed.
#5 Private Constructor
A private constructor in C# is a constructor that is only accessible within the class itself. It cannot be called from outside the class, which means that objects of the class cannot be created directly from outside the class. Private constructors are often used to prevent the instantiation of a class or to control the creation of objects through static factory methods within the class.
Here’s an example of a class with a private constructor:
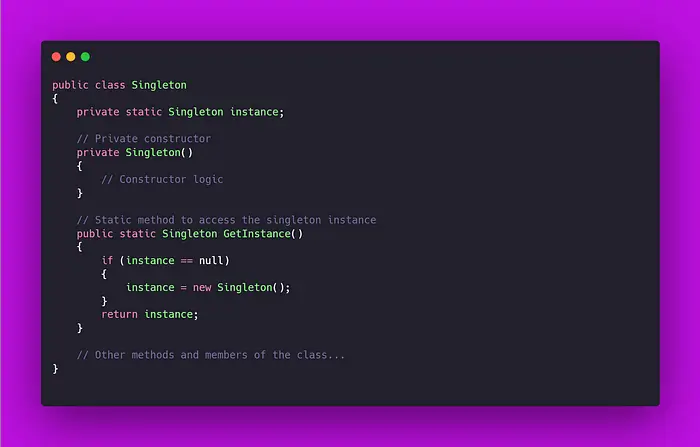
In this example, Singleton
is a class that implements the Singleton design pattern, ensuring that only one instance of the class is created. The constructor Singleton()
is marked as private, so it cannot be accessed from outside the class. Instead, the static method GetInstance()
is provided as a factory method to create or access the singleton instance. This method is responsible for controlling the creation of the singleton object and ensuring that only one instance is created throughout the lifetime of the application.
Aswe conclude our exploration of C# constructors, we’ve deep drive into the heart of object initialization, uncovering the versatility and power of constructors in crafting robust and efficient C# applications.
Throughout this journey, we’ve navigated through various types of constructors, from the humble default constructor to the dynamic parameterized constructor, and even the elusive copy constructor (or its equivalent) and the behind-the-scenes static constructor. Each type has its own unique role, offering flexibility, control, and efficiency in object creation and initialization.
By harnessing the capabilities of constructors, you’ve learned how to craft elegant and maintainable code, ensuring that your objects are initialized with precision and efficiency. Whether you’re creating simple data structures or complex object hierarchies, constructors provide the foundation upon which your code stands tall.
As you continue your C# development journey, remember the lessons learned here. Embrace constructors as your allies, wielding their power to create objects seamlessly, manage object lifecycles effectively, and enhance the clarity and readability of your codebase.
With a solid understanding of C# constructors and their myriad applications, you’re equipped to tackle real-world challenges with confidence and finesse. So go forth, fellow developer, and let the magic of constructors propel your C# projects to new heights.
Until next time, happy coding! ⚡