Welcome to our comprehensive guide on Object-Oriented Programming (OOP) using the C# programming language. In today’s rapidly evolving technological landscape, mastering OOP principles is essential for building scalable, maintainable, and efficient software solutions.
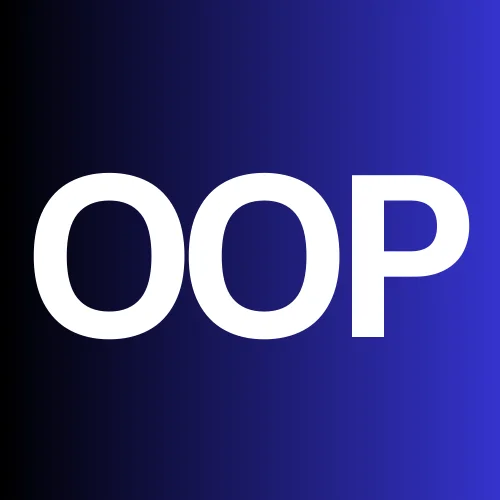
From the foundational building blocks of classes and objects to advanced topics like inheritance, polymorphism, and abstraction, this guide is designed to equip you with the knowledge and skills needed to leverage the full potential of OOP in your C# projects. Whether you’re a seasoned developer looking to refresh your understanding or a newcomer eager to learn, this resource aims to provide clear explanations, practical examples, and actionable insights to help you succeed in the world of OOP with C#.
C# is a versatile programming language developed by Microsoft, well-suited for implementing OOP concepts. Let’s explore some basic OOP principles and how they can be applied in C#:
#1 Classes and Objects:
Classes are blueprints for objects. They define the attributes (fields) and behaviors (methods) that objects of the class will have.
- In C#, you can define a class using the
class
keyword.
Consider a simple example of a Car
class:
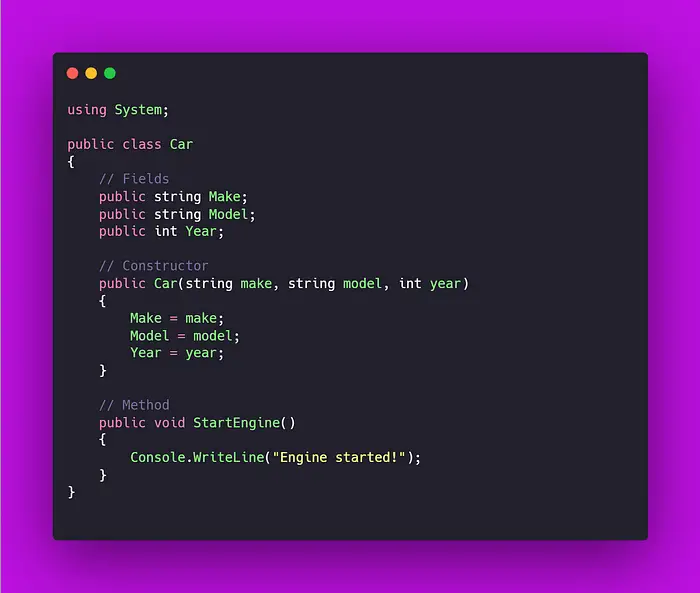
In this example, the Car
class has three fields (Make
, Model
, and Year
) representing the car’s attributes. It also has a constructor to initialize these fields and a method (StartEngine
) to start the car’s engine.
In C# OOP, an object is an instance of a class. When a class is defined, no memory is allocated, and no storage is reserved.
When an object is created from the class, memory is allocated and the object contains its own set of values for each instance variable defined in the class. An object is an instance of a class, and it can be manipulated using the methods and properties of that class. Objects can interact with each other, pass information back and forth, and perform actions based on the information they receive.
Now, let’s create an object of the Car
class and use it:
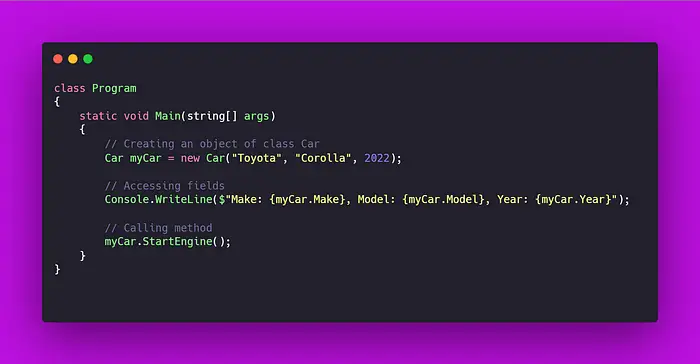
Output:
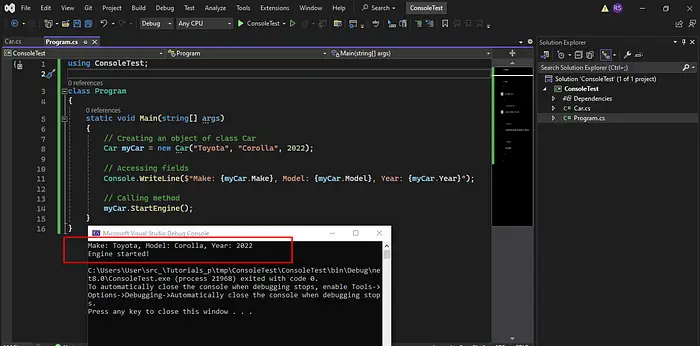
#2 Encapsulation:
Encapsulation means bundling the data (fields) and methods that operate on the data within a single unit (a class) and controlling access to that unit.
- In C#, you can achieve encapsulation by using access modifiers like
public
,private
,protected
, etc., to control access to class members.
Here is an example of encapsulation in C#:
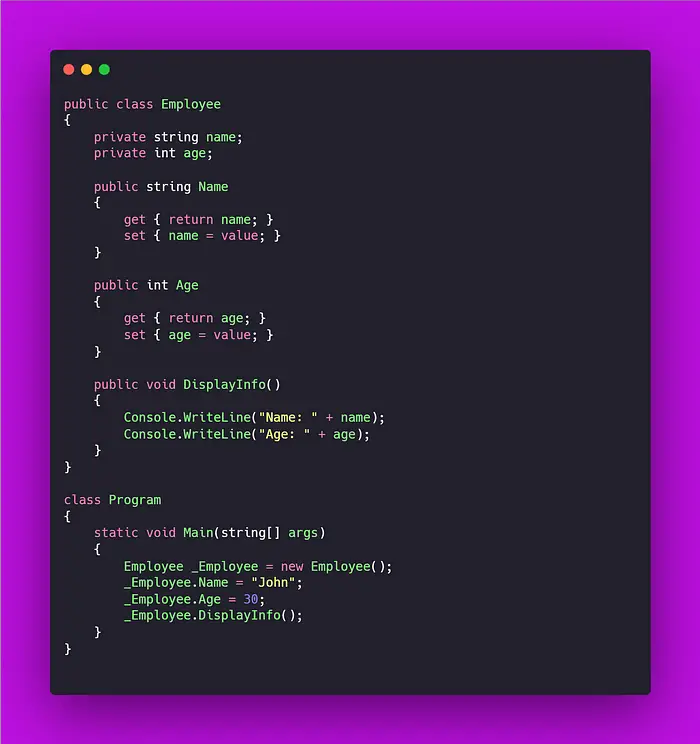
Output:
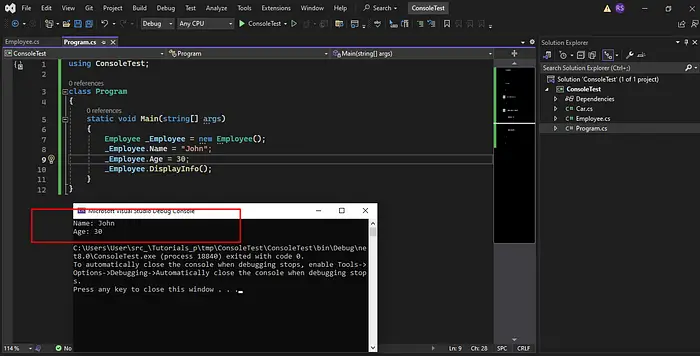
In this example, we have a class Employee
which has two private fields name
and age
. These fields are encapsulated by two public properties Name
and Age
, which provides access to the private fields using get
and set
methods. The DisplayInfo()
the method is a public method that displays the name
and age
of the employee.
In the Main()
method, we create an object of the Employee
class and set its Name
and Age
properties using the set
methods. We then call the DisplayInfo()
method to display employee information.
Encapsulation is demonstrated in this example by hiding the internal implementation details of the Employee
class (the private fields) and providing access to them through public properties.
#3 Inheritance:
Inheritance is a mechanism where a new class (derived class) is created from an existing class (base class), inheriting its attributes and behaviors.
- In C#, you can implement inheritance using the
: baseClass
syntax. For example:
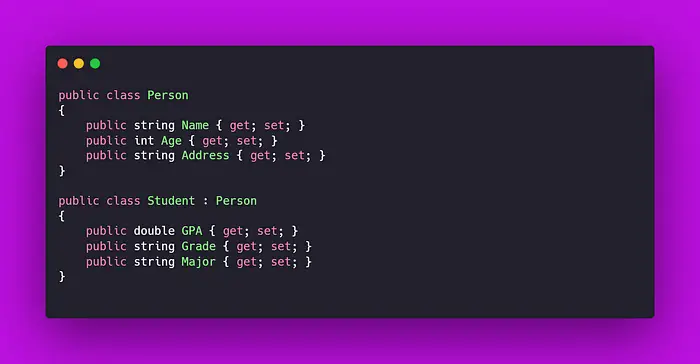
In this example, the Student
class inherits from the Person
class using the :
symbol. The Student
class adds its properties such as GPA
, Grade
, and Major
, and has access to the properties and methods of the Person
class such as Name
, Age
, and Address
.
#4 Polymorphism:
Polymorphism allows objects of different classes to be treated as objects of a common superclass.
- In C#, polymorphism is achieved through method overriding and method overloading.
Here’s an example of polymorphism in C#:
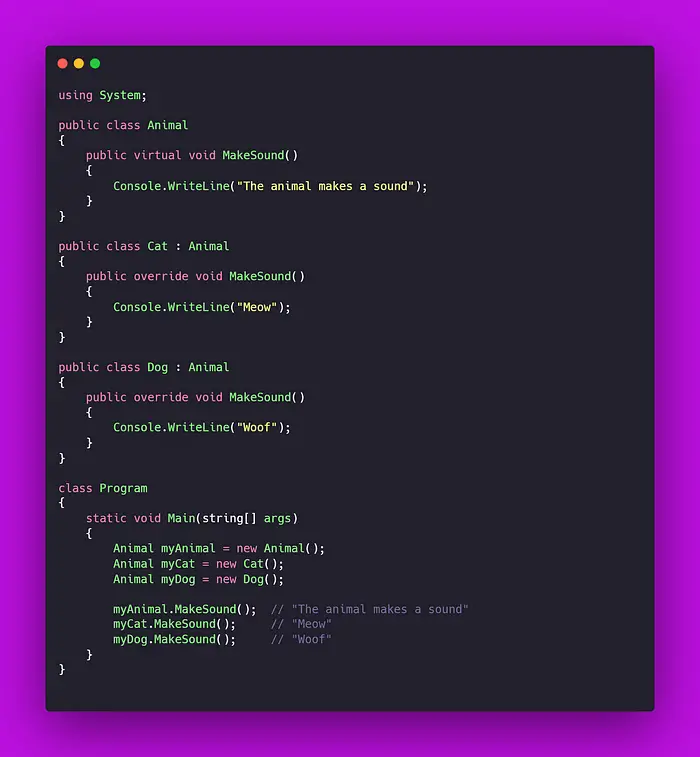
In this example, we have a base Animal
class with a MakeSound
method that we mark as virtual
, which means it can be overridden in derived classes. We then create two derived classes, Cat
and Dog
, which overrides the MakeSound
method with their specific implementations. Finally, in the Main
method, we create instances of each class and call the MakeSound
method on each one. Because of polymorphism, each instance will call its implementation of MakeSound
, even though the variable type is Animal
.
#5 Abstraction:
Abstraction involves hiding the complex implementation details and showing only the essential features of the object.
- In C#, you can achieve abstraction using abstract classes and interfaces.
Here’s an example of abstraction in C#:
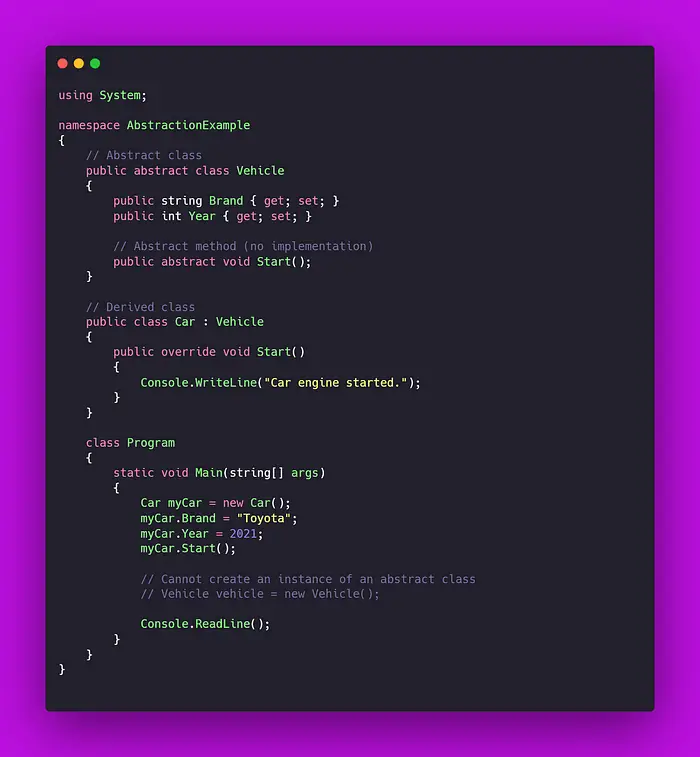
In this example, we have an abstract class Vehicle
that has two properties Brand
and Year
and an abstract method Start()
. The Start()
method does not have any implementation, but we know that all vehicles can start, so it is declared as an abstract method.
We then have a derived class Car
that inherits from the Vehicle
class and overrides the Start()
method with its own implementation. In the Main
method, we create an instance of the Car
class and call the Start()
method.
Note that we cannot create an instance of an abstract class, so we cannot create an instance of the Vehicle
class.
#6 Interfaces:
Interfaces define a contract that implementing classes must adhere to. They specify the methods and properties that a class must implement.
- In C#, you can declare an interface using the
interface
keyword.
Here’s an example of an interface in C#:
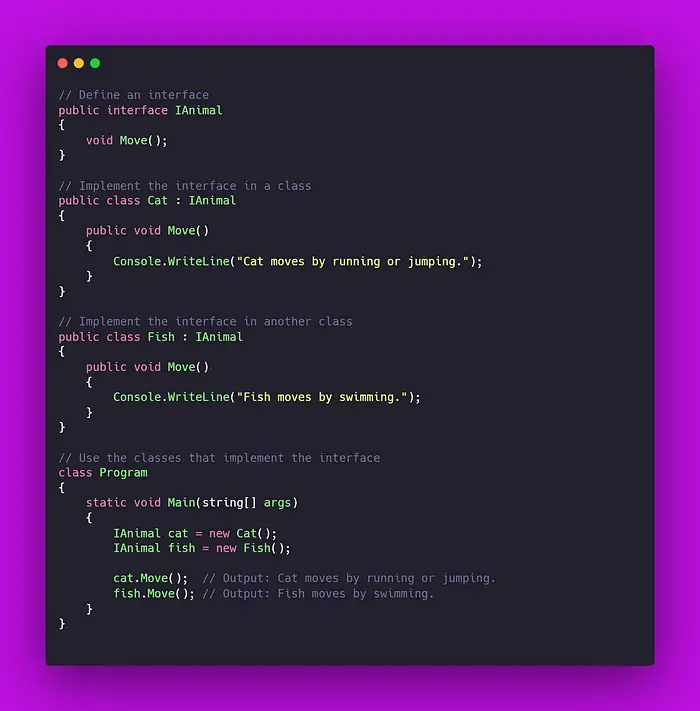
In the Main
method, instances of Cat
and Fish
are created, but they are declared as IAnimal
types, which means they can hold any object that implements the IAnimal
interface.
These are some of the fundamental principles of OOP, and C# provides robust support for implementing them. By applying these principles effectively, you can create well-structured, maintainable, and scalable C# applications.
Summary:
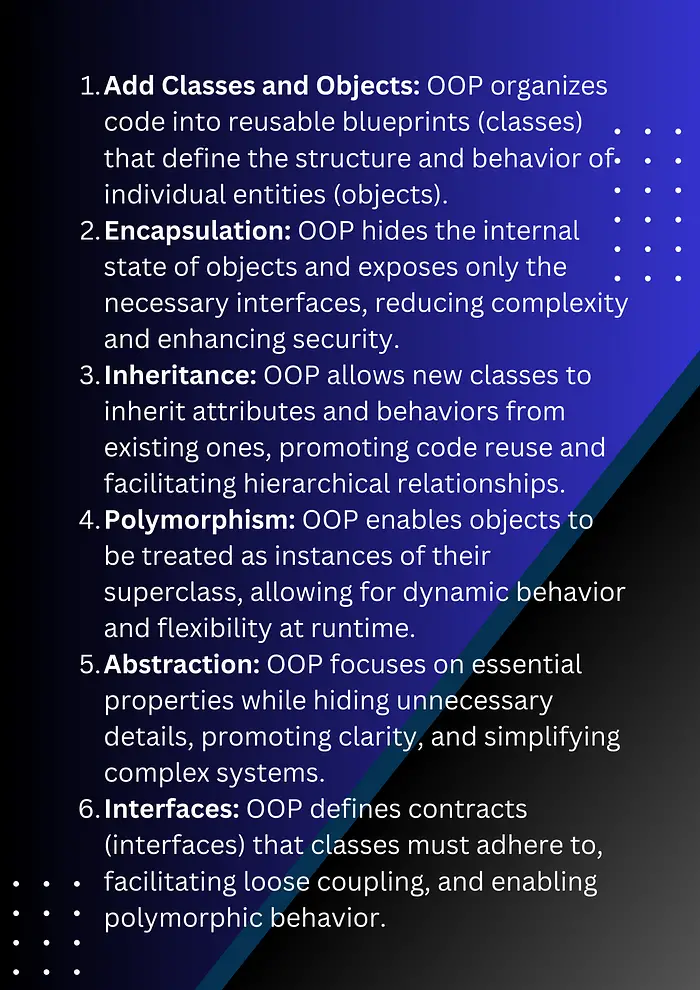
Aswe conclude our exploration of Object-Oriented Programming (OOP) using the C# programming language, it’s clear that OOP is not just a programming paradigm but a powerful mindset that shapes the way we design and develop software. Throughout this guide, we’ve uncovered the fundamental principles of OOP — classes, objects, encapsulation, inheritance, polymorphism, abstraction, and interfaces — and witnessed how they come to life in the world of C#.
By mastering OOP concepts in C#, you gain the ability to create code that is modular, reusable, and easy to maintain. You unlock the potential to build complex systems with clear structure and logical organization, enabling collaboration among developers and facilitating the evolution of your codebase over time.
However, our journey doesn’t end here. Object-Oriented Programming is a vast topic with endless opportunities for exploration and growth. As you continue your learning journey, remember to practice, experiment, and apply these concepts in your own projects. Embrace challenges, seek feedback, and strive for continuous improvement.
Whether you’re developing desktop applications, web services, mobile apps, or games, OOP principles will serve as your guiding light, empowering you to write elegant, efficient, and scalable code in C#. So, go forth with confidence, harness the power of OOP, and embark on new adventures in software development.
Thank you for joining us on this enlightening journey through Object-Oriented Programming with C#. May your code be clean, your designs be elegant, and your projects be successful.
Happy coding!