Accessing APIs is a fundamental aspect of modern web development, enabling applications to communicate and exchange data seamlessly across different platforms and services. In ASP.NET Web API development, ensuring secure and authenticated access to APIs is paramount. One common method to achieve this is by utilizing API keys. These keys serve as unique identifiers, granting authorized access to specific APIs while safeguarding against unauthorized usage.
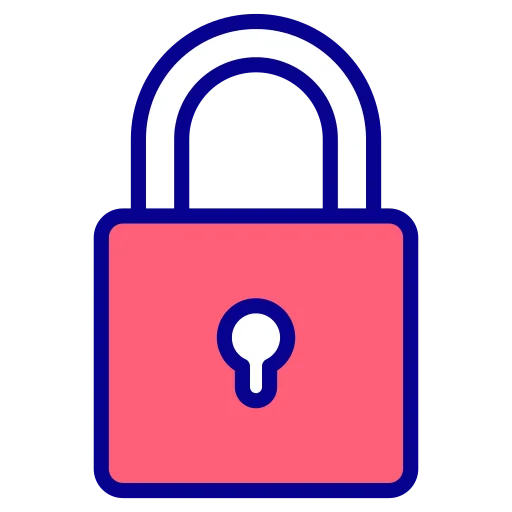
In this guide, we will explore how to effectively access APIs using API keys within the context of ASP.NET Web API. By implementing key validation mechanisms, developers can regulate access to their APIs, ensuring data integrity, confidentiality, and adherence to security best practices. Through a combination of configuration settings and custom message handlers, ASP.NET Web API empowers developers to seamlessly integrate API key authentication into their applications, fostering a secure and robust API ecosystem. Let’s jump into the intricacies of implementing API key authentication in ASP.NET Web API, equipping developers with the knowledge and tools necessary to enhance the security and reliability of their web applications.
To access an API using a key in ASP.NET Web API, you typically need to pass the API key either in the request headers or as a query parameter. Here’s a basic guide on how to do it:
#1 Create Web API Project with .NET 8.0
I’m considering you already have a web API project. If the project connected with the MSSQL database then awesome!
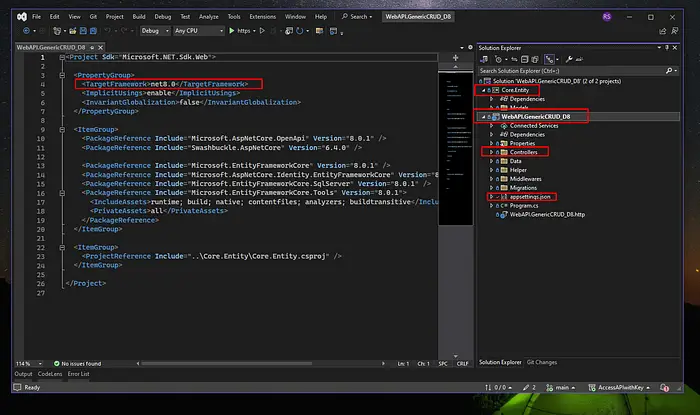
#2 Generate Or Set an API Key:
Before you can access the API, you’ll need to generate an API key. This key acts as a token to authenticate your requests. You can set the key in the appsetting.json file.
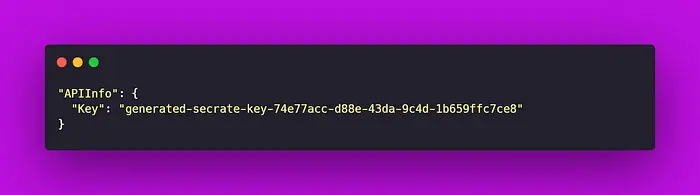
#3 Create Middleware | Set Up API Key Validation:
In your ASP.NET Web API project, you’ll need to create middleware for validation. This usually involves creating a custom message handler to intercept incoming requests and validate the API key.
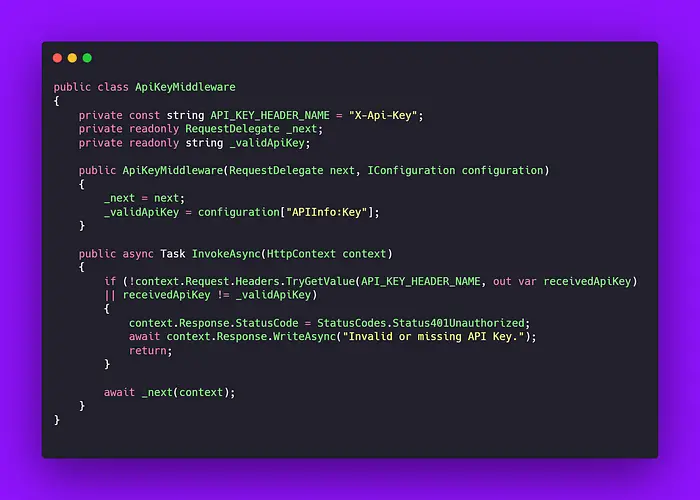
#4 Add Middleware in Program.cs
app.UseMiddleware<ApiKeyMiddleware>();
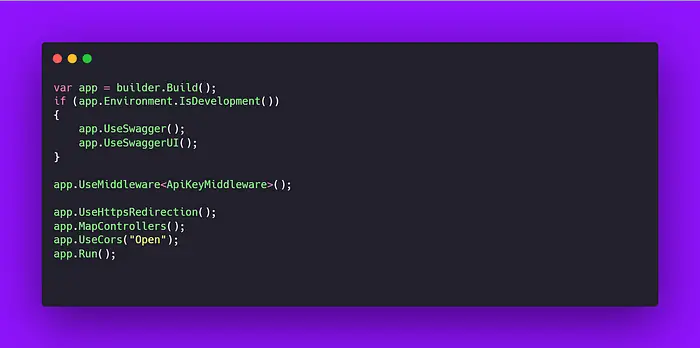
#5 Pass API Key in Request Headers:
Modify your client application to pass the API key in the request headers. This involves adding an “Authorization” header with the API key value.
url: 'http://localhost:5004/api/Branch/GetAll',
type: 'GET',
beforeSend: function(xhr) {
xhr.setRequestHeader('x-api-key', apiKey);
},
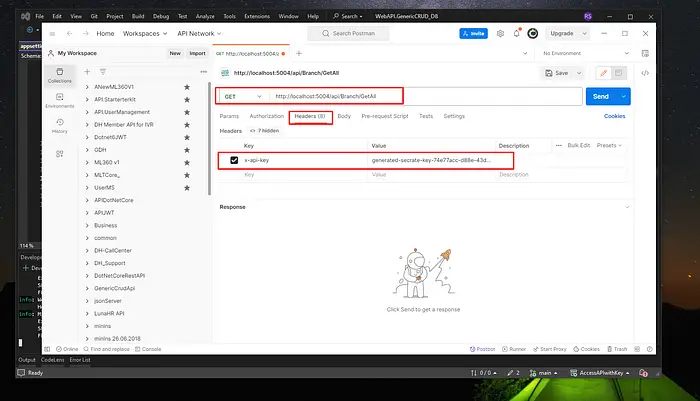
#6 Access the API: Using jQuery
To call the APIÂ http://localhost:5004/api/Branch/GetAll
 using a jQuery AJAX call with the x-api-key
 header, you can use the following code:
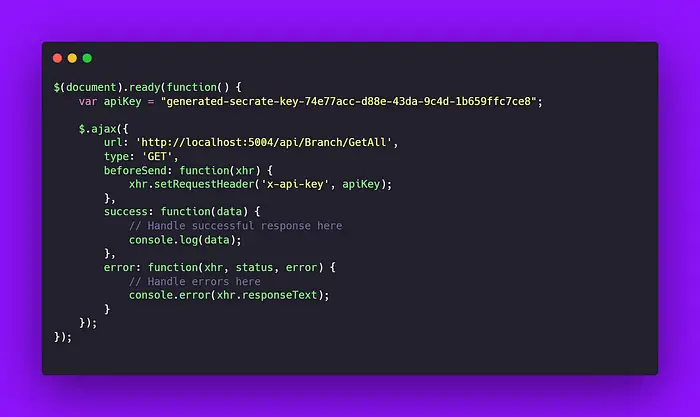
#7 Access the API: Using Postman
Once the API key validation is set up correctly, you can make requests to the API from your client application, and the ASP.NET Web API will validate the API key before processing the request.
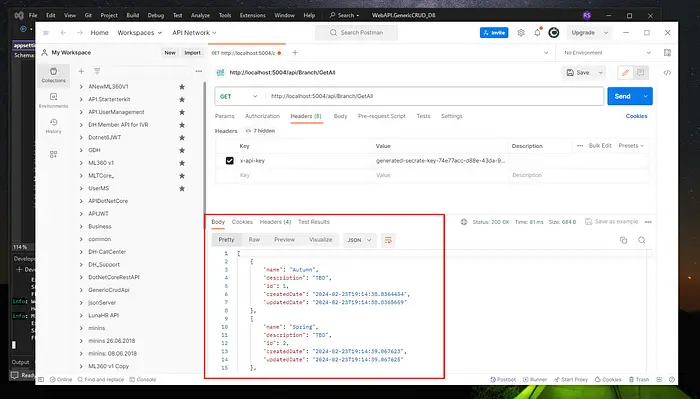
Inconclusion, mastering the art of accessing APIs with keys using ASP.NET Web API is pivotal for ensuring the security and integrity of web applications. By incorporating API key authentication mechanisms, developers can fortify their APIs against unauthorized access and potential security threats. The implementation of custom message handlers and configuration settings empowers developers to seamlessly integrate API key validation into their ASP.NET Web API projects, promoting a secure and efficient exchange of data between clients and servers.
Furthermore, leveraging API keys not only enhances security but also facilitates better management of API usage, enabling developers to monitor and control access to their services effectively. As the digital landscape continues to evolve, prioritizing API security remains essential in safeguarding sensitive information and preserving the trust of users and stakeholders.
By following the guidelines outlined in this guide, developers can harness the full potential of ASP.NET Web API to create robust, secure, and scalable web applications that meet the demands of today’s interconnected world. With a solid understanding of API key authentication and implementation techniques, developers can navigate the complexities of web development with confidence, delivering exceptional experiences for users while maintaining the highest standards of security and reliability.
đź‘‹ .NET Application Collections
🚀 My Youtube Channel
đź’» Github | Full Project