ASP.NET MVC, combined with the power of Dapper Micro ORM and leveraging the capabilities of .NET 8.0, offers a robust and efficient solution for building web applications. In this exploration, we explore the details of Generic CRUD (Create, Read, Update, Delete) operations using Dapper, a lightweight and high-performance micro ORM designed for .NET.
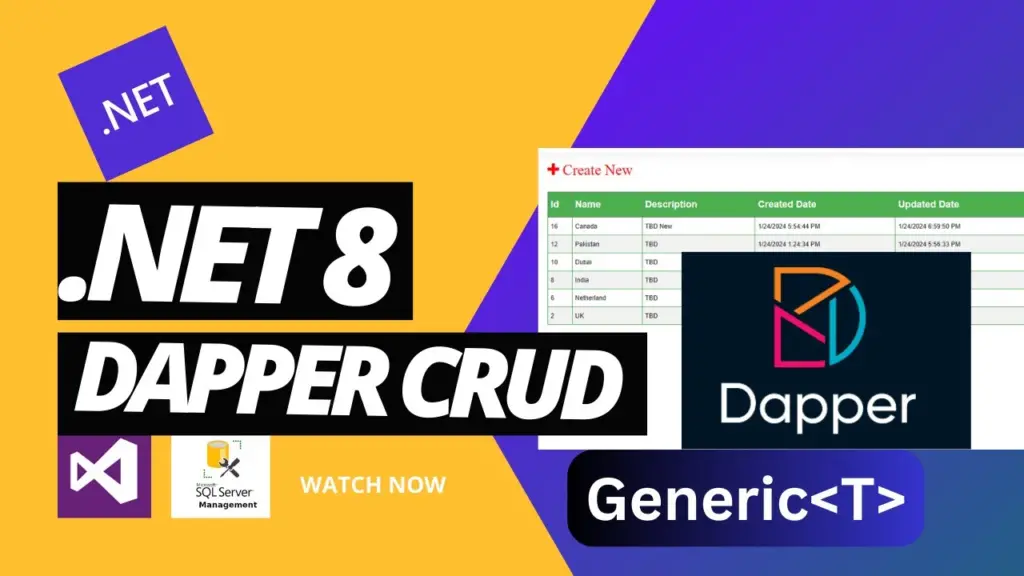
The integration of ASP.NET MVC with Dapper provides a flexible and scalable approach to database interactions, allowing developers to streamline data access without sacrificing performance. With the enhancements introduced in .NET 8.0, this combination delivers a modern and feature-rich development experience for building dynamic and data-driven web applications. Join us on this journey as we showcase the implementation of generic CRUD operations in an ASP.NET MVC application, demonstrating the collaboration between Dapper and the latest features of .NET 8.0.
Creating a project for ASP.NET MVC with Dapper Micro ORM and .NET 8.0 involves several steps. Below are the essential steps to set up a project:
1# Create a New Project Using Visual Studio
Ensure that you have Visual Studio installed on your machine. You can download the latest version from the official Visual Studio website.
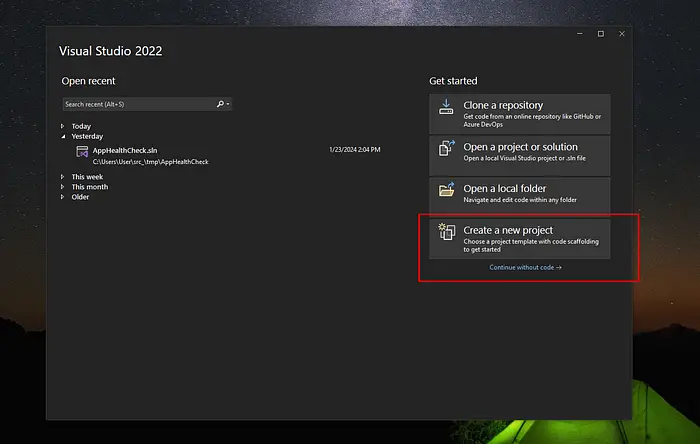
- Create a New ASP.NET MVC Project: Open Visual Studio and create a new project.
- Select “Create a new project.”
- Choose “Web Application (Model-View-Controller)” as the project template.
- Select the ASP.NET Core version compatible with .NET 8.0.
- Click “Create” to generate the project structure.
- Make sure you already installed .NET SDK 8.0
dotnet --version
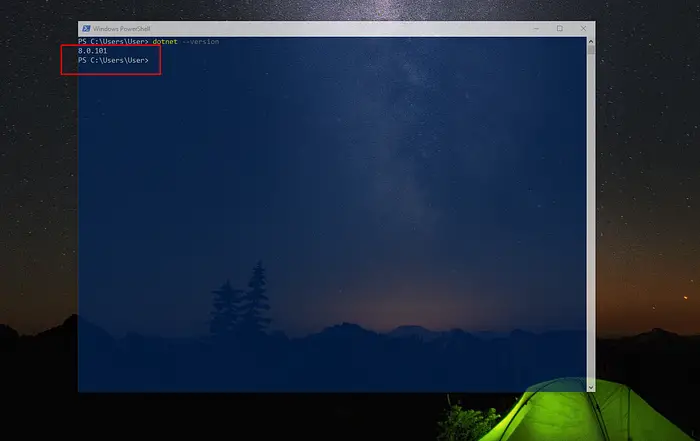
2# Install Dapper and System.Data.SqlClient Packages:
In your ASP.NET MVC project, install the necessary NuGet packages for Dapper and SQL Server data access:
dotnet add package Microsoft.Data.SqlClient --version 5.1.2
dotnet add package Dapper.Extensions.NetCore --version 5.1.4
dotnet add package Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation --version 8.0.1
3# Create Model Class:
Define your model class representing the entity you want to perform CRUD operations. For example:
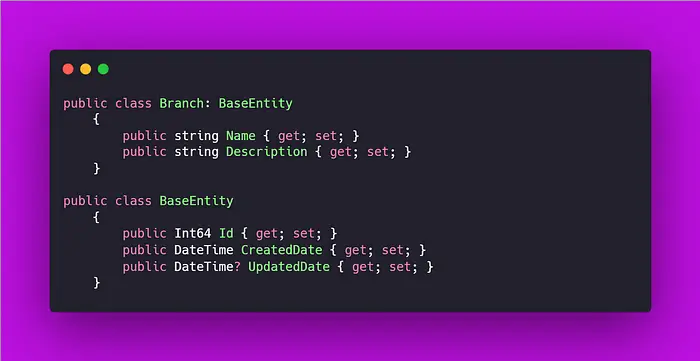
public class Branch: BaseEntity
{
public string Name { get; set; }
public string Description { get; set; }
}
public class BaseEntity
{
public Int64 Id { get; set; }
public DateTime CreatedDate { get; set; }
public DateTime? UpdatedDate { get; set; }
}
4.1 # Create a Generic Repository Class:
Create and Implement a generic repository class that uses Dapper for database operations.
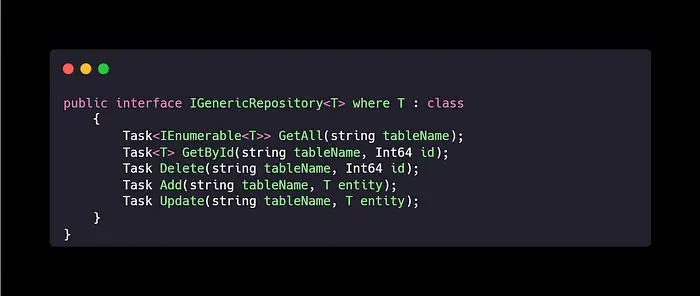
4.2# Implement Generic Repository Class
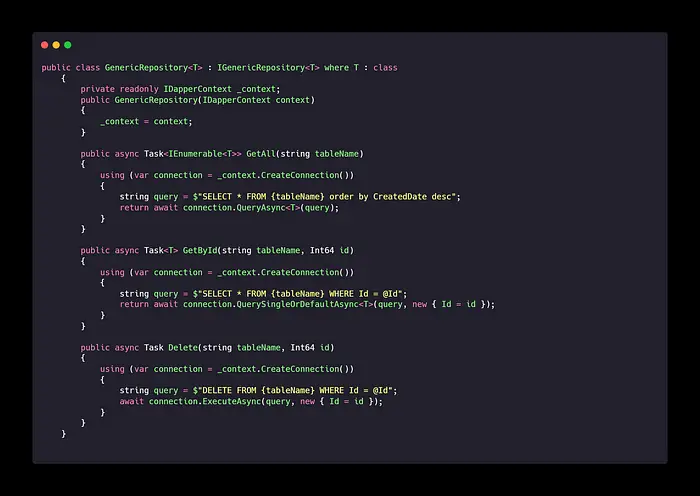
5# Configure Database Connection:
Set up the connection string in your appsettings.json
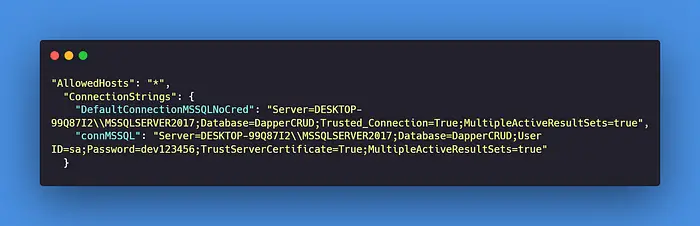
"AllowedHosts": "*",
"ConnectionStrings": {
"DefaultConnectionMSSQLNoCred": "Server=DESKTOP-99Q87I2\\MSSQLSERVER2017;Database=DapperCRUD;Trusted_Connection=True;MultipleActiveResultSets=true",
"connMSSQL": "Server=DESKTOP-99Q87I2\\MSSQLSERVER2017;Database=DapperCRUD;User ID=sa;Password=dev123456;TrustServerCertificate=True;MultipleActiveResultSets=true"
}
6# Configure Dependency Injection:
Register your repository class for dependency injection in the Program.cs
file.
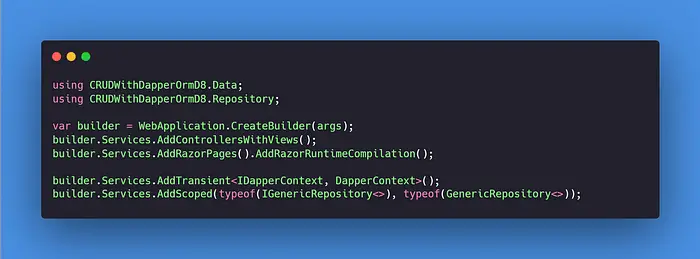
builder.Services.AddTransient<IDapperContext, DapperContext>();
builder.Services.AddScoped(typeof(IGenericRepository<>), typeof(GenericRepository<>));
7# Create Controllers and Views:
Create controllers and corresponding views for your entity. Implement actions for listing, creating, updating, and deleting records. Use the generic repository to perform CRUD operations.
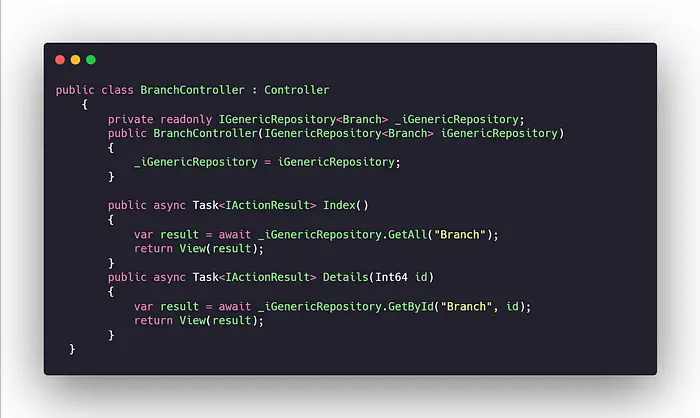
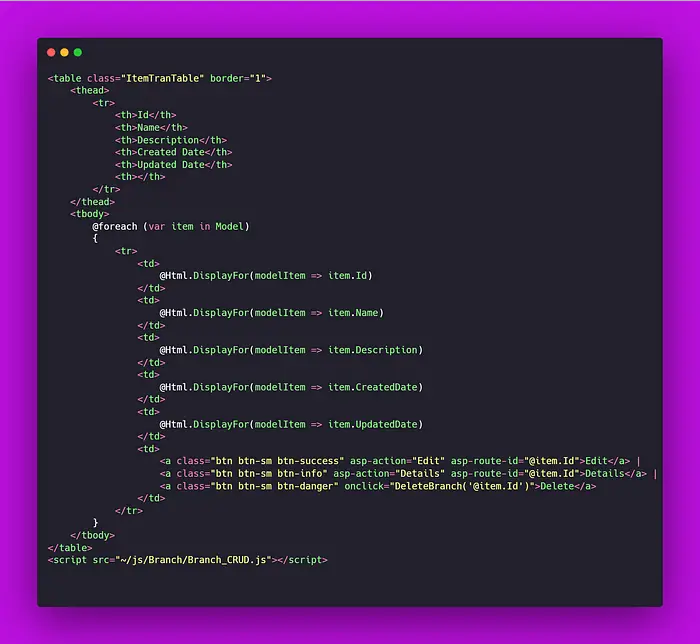
8# Run and Test:
Build and run your ASP.NET MVC application. Test the generic CRUD operations by navigating to the relevant views and verifying that data is correctly retrieved, added, updated, and deleted.
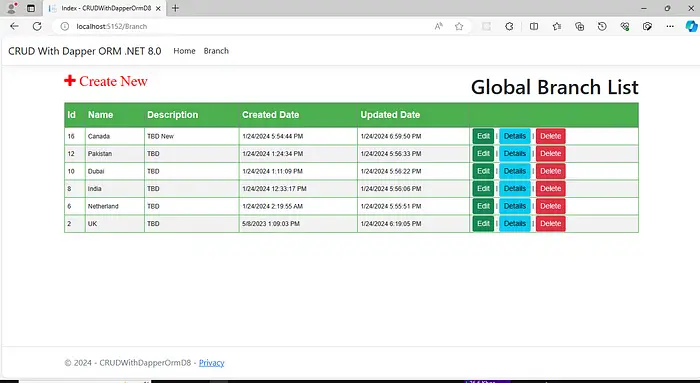
Following these steps, you’ll have a basic ASP.NET MVC project using Dapper Micro ORM and .NET 8.0, with a generic repository for performing CRUD operations on a specific entity. Adjust the code based on your specific requirements and database structure.
Full Project Video Explanation
In the area of web development, the combination of ASP.NET MVC, Dapper Micro ORM, and the cutting-edge capabilities of .NET 8.0 has proven to be a formidable toolkit for building dynamic and efficient web applications. Throughout this exploration, we embarked on a journey to implement a Generic CRUD (Create, Read, Update, Delete) system, showcasing the seamless integration of Dapper with ASP.NET MVC and leveraging the features introduced in .NET 8.0.
The use of Dapper, a lightweight micro ORM, demonstrated its prowess in simplifying database interactions while maintaining high performance. By adopting a generic repository pattern, developers can achieve a flexible and scalable approach to data access, promoting code reusability and maintainability. The marriage of ASP.NET MVC and Dapper not only facilitates smooth integration with databases but also enhances the development experience by providing a clean and intuitive syntax.
The advancements in .NET 8.0 further elevate the development process, offering modern features and improvements that contribute to the overall robustness of the application. From enhanced performance to new language features, .NET 8.0 empowers developers to build web applications that are not only feature-rich but also aligned with the latest industry standards.
As we conclude our exploration of ASP.NET MVC Generic CRUD with Dapper Micro ORM in the context of .NET 8.0, we’ve witnessed the synergy between these technologies, offering a compelling solution for developers seeking an efficient and maintainable approach to web application development. By harnessing the strengths of each component, developers can create web applications that are not only powerful but also adaptive to the evolving landscape of technology. The journey continues, beckoning developers to explore and innovate in the ever-evolving ecosystem of web development with ASP.NET, Dapper, and .NET 8.0.
Happy Coding!