When diving into C# .NET development, efficiency and productivity are key. Whether you’re a seasoned developer or just starting, having a collection of useful snippets at your fingertips can significantly enhance your workflow. These snippets not only save time but also help in writing clean, efficient, and bug-free code.
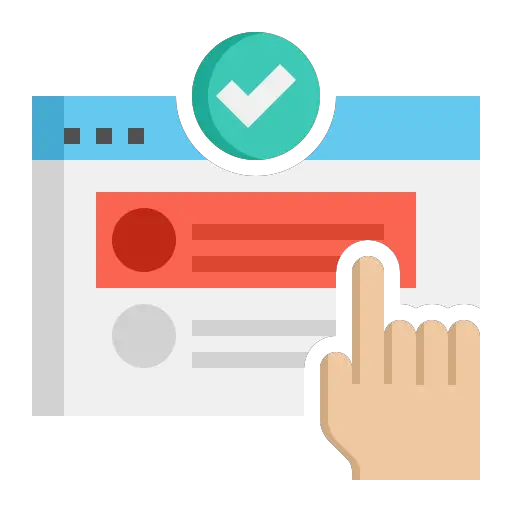
In this blog post, we’ll explore some of the most useful C# .NET snippets that can aid in everyday development tasks. From basic operations like string manipulation and file handling to more advanced tasks such as JSON serialization, LINQ queries, and asynchronous programming, these snippets will prove invaluable. So, let’s dive in and supercharge your C# .NET coding experience! 🚀
Why Use Code Snippets?
Code snippets are pre-defined blocks of code that can be easily inserted into your programs. They serve several purposes:
- Boost Productivity:Â By reusing common code patterns, you can focus more on the logic and less on repetitive tasks.
- Ensure Consistency:Â Snippets help maintain coding standards and consistency across different projects.
- Reduce Errors:Â Reusing tested snippets minimizes the chance of introducing bugs into your code.
The Collection
Here’s a list of the top C# .NET snippets that every developer should have in their toolkit. These snippets cover a range of common scenarios you are likely to encounter:
#1 Check if String is Null or Empty
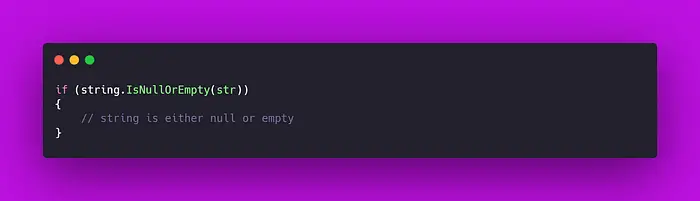
if (string.IsNullOrEmpty(str))
{
// string is either null or empty
}
#2 Convert String to Integer (with error handling)
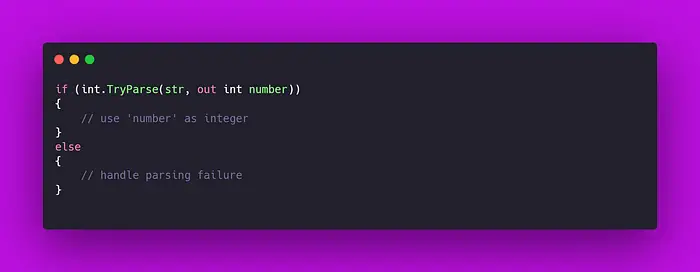
if (int.TryParse(str, out int number))
{
// use 'number' as integer
}
else
{
// handle parsing failure
}
#3 Convert Object to JSON String
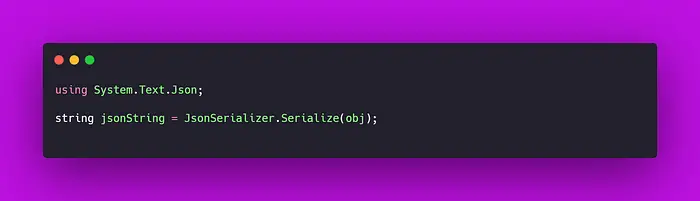
using System.Text.Json;
string jsonString = JsonSerializer.Serialize(obj);
#4 Deserialize JSON String to Object
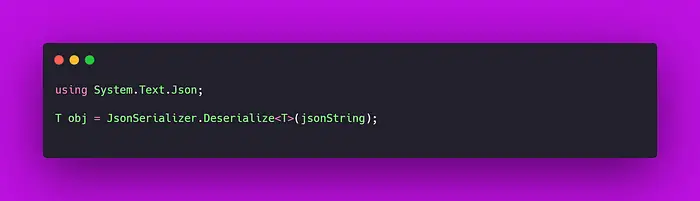
using System.Text.Json;
T obj = JsonSerializer.Deserialize<T>(jsonString);
#5 Read the Entire Text File into String
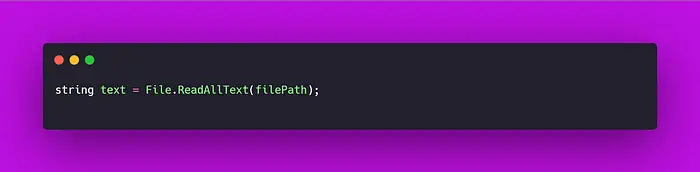
string text = File.ReadAllText(filePath);
#6 Write String to Text File
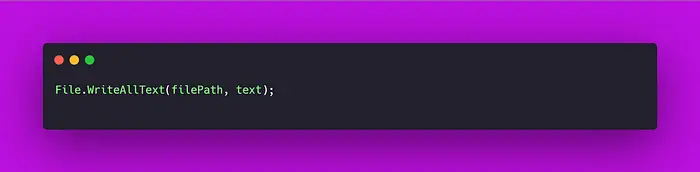
File.WriteAllText(filePath, text);
#7 LINQ Query Example
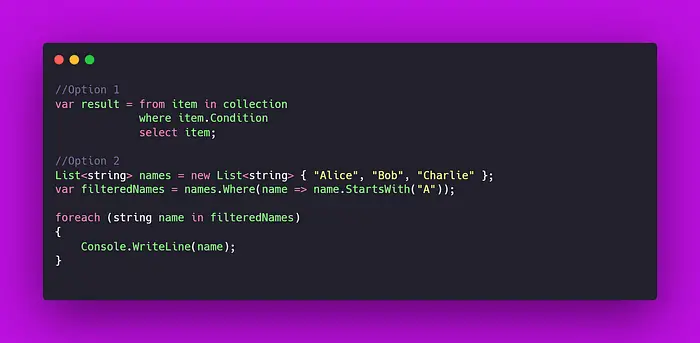
//Option 1
var result = from item in collection
where item.Condition
select item;
//Option 2
List<string> names = new List<string> { "Alice", "Bob", "Charlie" };
var filteredNames = names.Where(name => name.StartsWith("A"));
foreach (string name in filteredNames)
{
Console.WriteLine(name);
}
#8 DateTime Formatting
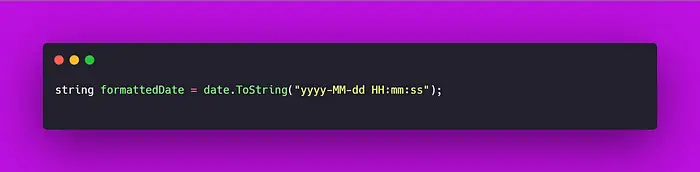
string formattedDate = date.ToString("yyyy-MM-dd HH:mm:ss");
#9 Using HttpClient
 to Make a GET Request
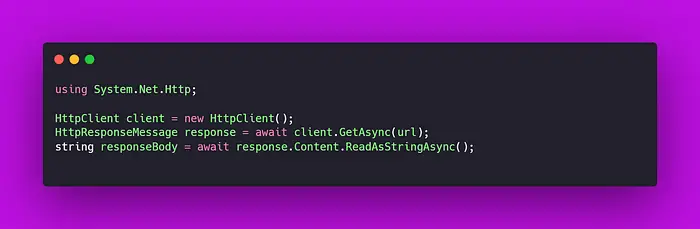
using System.Net.Http;
HttpClient client = new HttpClient();
HttpResponseMessage response = await client.GetAsync(url);
string responseBody = await response.Content.ReadAsStringAsync();
#10 Simple Multithreading with Task
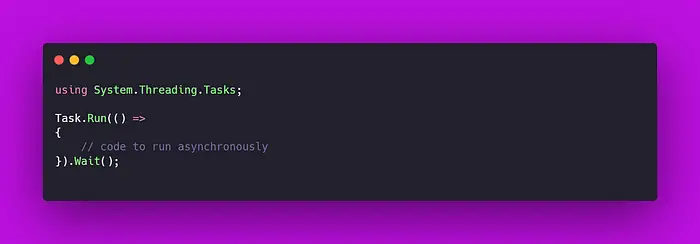
using System.Threading.Tasks;
Task.Run(() =>
{
// code to run asynchronously
}).Wait();
#11 Object Initialization Syntax
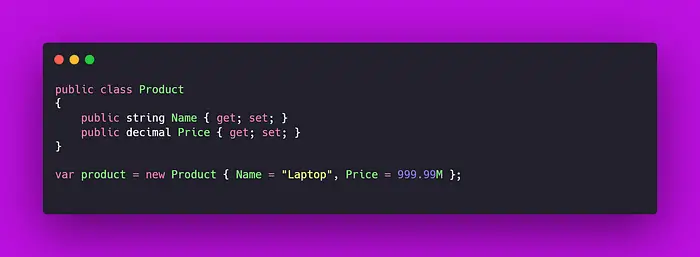
public class Product
{
public string Name { get; set; }
public decimal Price { get; set; }
}
var product = new Product { Name = "Laptop", Price = 999.99M };
#12 Enumerable.Range Method
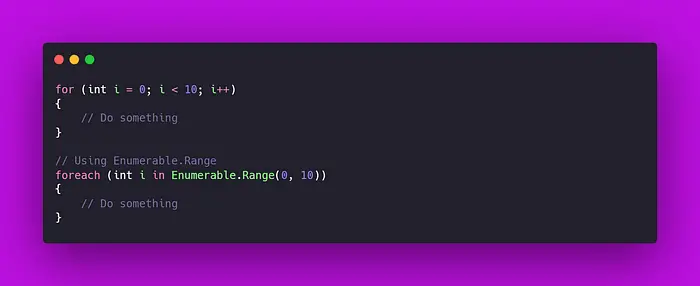
for (int i = 0; i < 10; i++)
{
// Do something
}
// Using Enumerable.Range
foreach (int i in Enumerable.Range(0, 10))
{
// Do something
}
#13 Conditional Ternary Operator
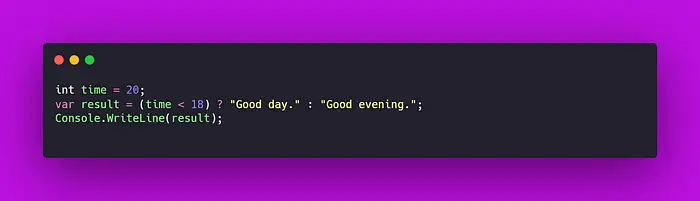
int time = 20;
var result = (time < 18) ? "Good day." : "Good evening.";
Console.WriteLine(result);
#14 String Interpolation
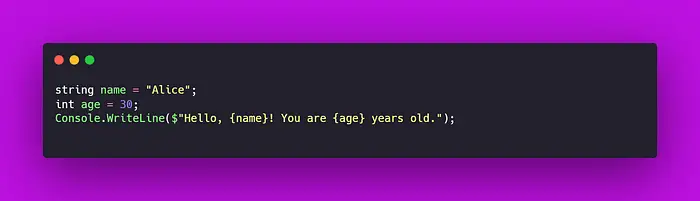
string name = "Alice";
int age = 30;
Console.WriteLine($"Hello, {name}! You are {age} years old.");
#15 Using Statement
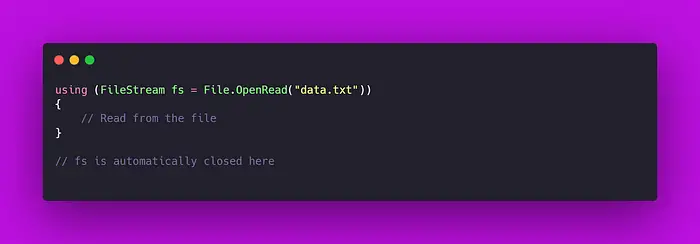
using (FileStream fs = File.OpenRead("data.txt"))
{
// Read from the file
}
// fs is automatically closed here
#16 Expression-Bodied Members
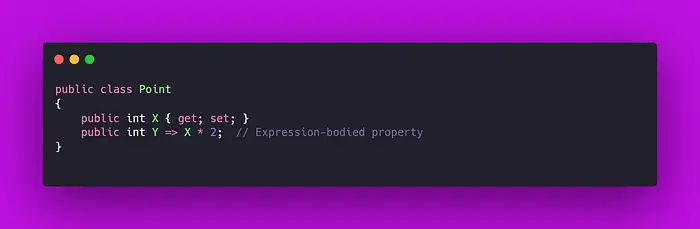
public class Point
{
public int X { get; set; }
public int Y => X * 2; // Expression-bodied property
}
#17 Dictionary Initialization
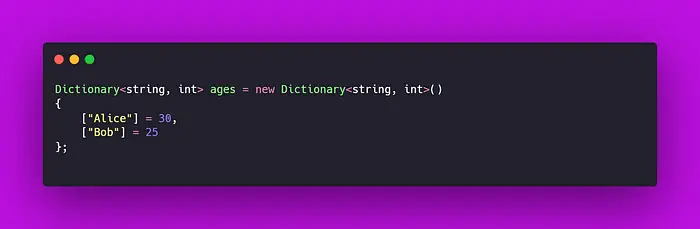
Dictionary<string, int> ages = new Dictionary<string, int>()
{
["Alice"] = 30,
["Bob"] = 25
};
#18Â Reflection
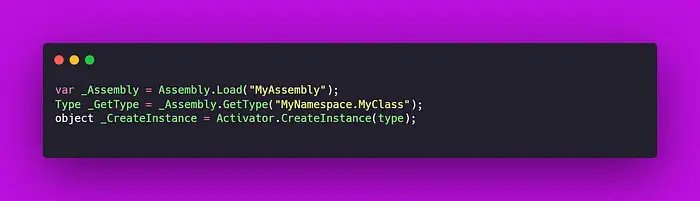
var _Assembly = Assembly.Load("MyAssembly");
Type _GetType = _Assembly.GetType("MyNamespace.MyClass");
object _CreateInstance = Activator.CreateInstance(type);
#19 Commenting Code
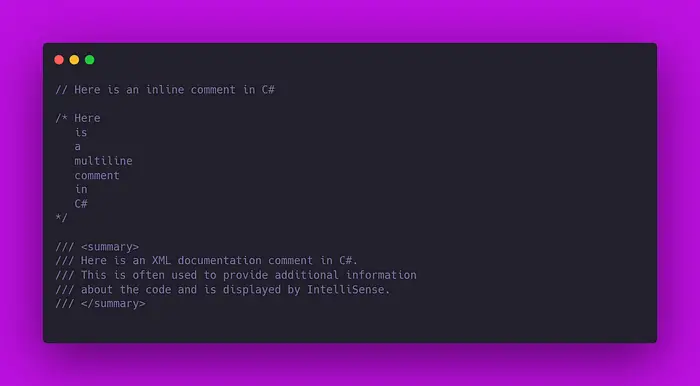
// Here is an inline comment in C#
/* Here
is
a
multiline
comment
in
C#
*/
/// <summary>
/// Here is an XML documentation comment in C#.
/// This is often used to provide additional information
/// about the code and is displayed by IntelliSense.
/// </summary>
#20 Testing Performance Using Stopwatch
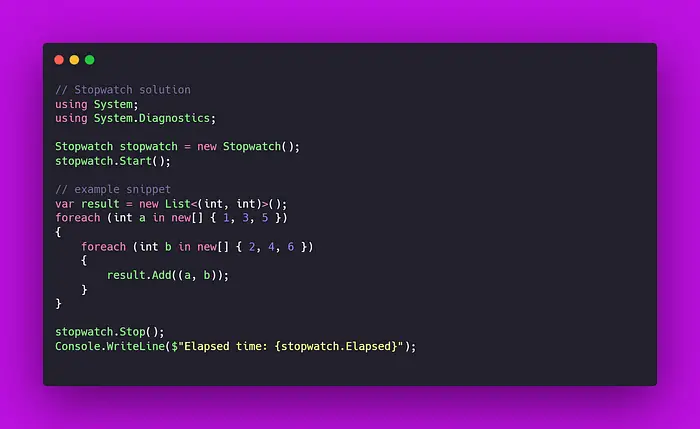
// Stopwatch solution
using System;
using System.Diagnostics;
Stopwatch stopwatch = new Stopwatch();
stopwatch.Start();
// example snippet
var result = new List<(int, int)>();
foreach (int a in new[] { 1, 3, 5 })
{
foreach (int b in new[] { 2, 4, 6 })
{
result.Add((a, b));
}
}
stopwatch.Stop();
Console.WriteLine($"Elapsed time: {stopwatch.Elapsed}");
#21 Using Finally Block
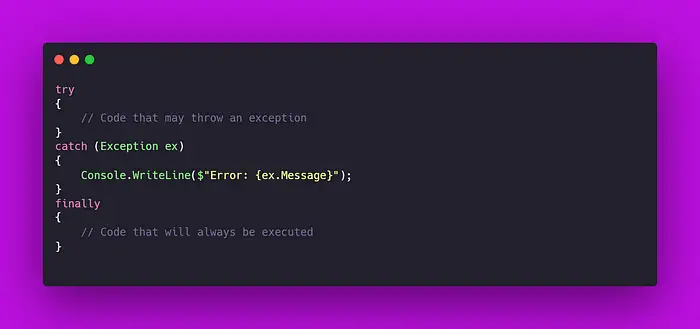
try
{
// Code that may throw an exception
}
catch (Exception ex)
{
Console.WriteLine($"Error: {ex.Message}");
}
finally
{
// Code that will always be executed
}
By incorporating these snippets into your C# code, you’ll enhance readability, maintainability, and efficiency in your .NET applications.
These snippets are designed to be easy to understand and integrate into your projects. They address some of the most frequent coding challenges and provide elegant solutions that you can rely on.
Having a repertoire of reliable and efficient C# .NET snippets can dramatically enhance your development productivity. These snippets serve as quick solutions to common problems, allowing you to focus more on the creative aspects of coding rather than the repetitive ones. Whether it’s handling strings, performing file operations, working with JSON, or managing asynchronous tasks, these snippets cover a broad range of functionalities that are indispensable in everyday programming.
By integrating these snippets into your workflow, you’ll not only write cleaner and more consistent code but also reduce the likelihood of errors and bugs. Keep this collection handy, and you’ll find yourself coding faster and smarter, ready to tackle any challenge that comes your way.
Happy coding! 🚀
đź‘‹ .NET Application Collections
🚀 My Youtube Channel
đź’» Github